Conditionals
Code blocks used in this tutorial:
Variables
In programming, we use variables to keep track of numbers and states. You can think of variables like boxes with labels.
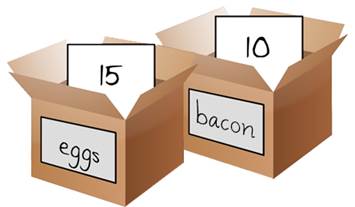
Electronics
For this tutorial, we are going to use the expansion pack
, the LED module
and 3 cables.
Let’s do the following steps:
- Grab 1 cable and connect the
S
pin of theLED module
to theS0
pin of theexpansion pack
. Make sure that the cable is connected to theS0
position. - Next, grab another cable and connect the
+
pin of theLED module
to theV1
pin of theexpansion pack
. This can be connected on any pin as long as it is a red pin ofV1
. - Finally, grab the last cable and connect the
-
pin of theLED module
to theG
pin of theexpansion pack
. This can be connected on any pin as long as it is a black pin ofG
.
You should have something like the picture below:
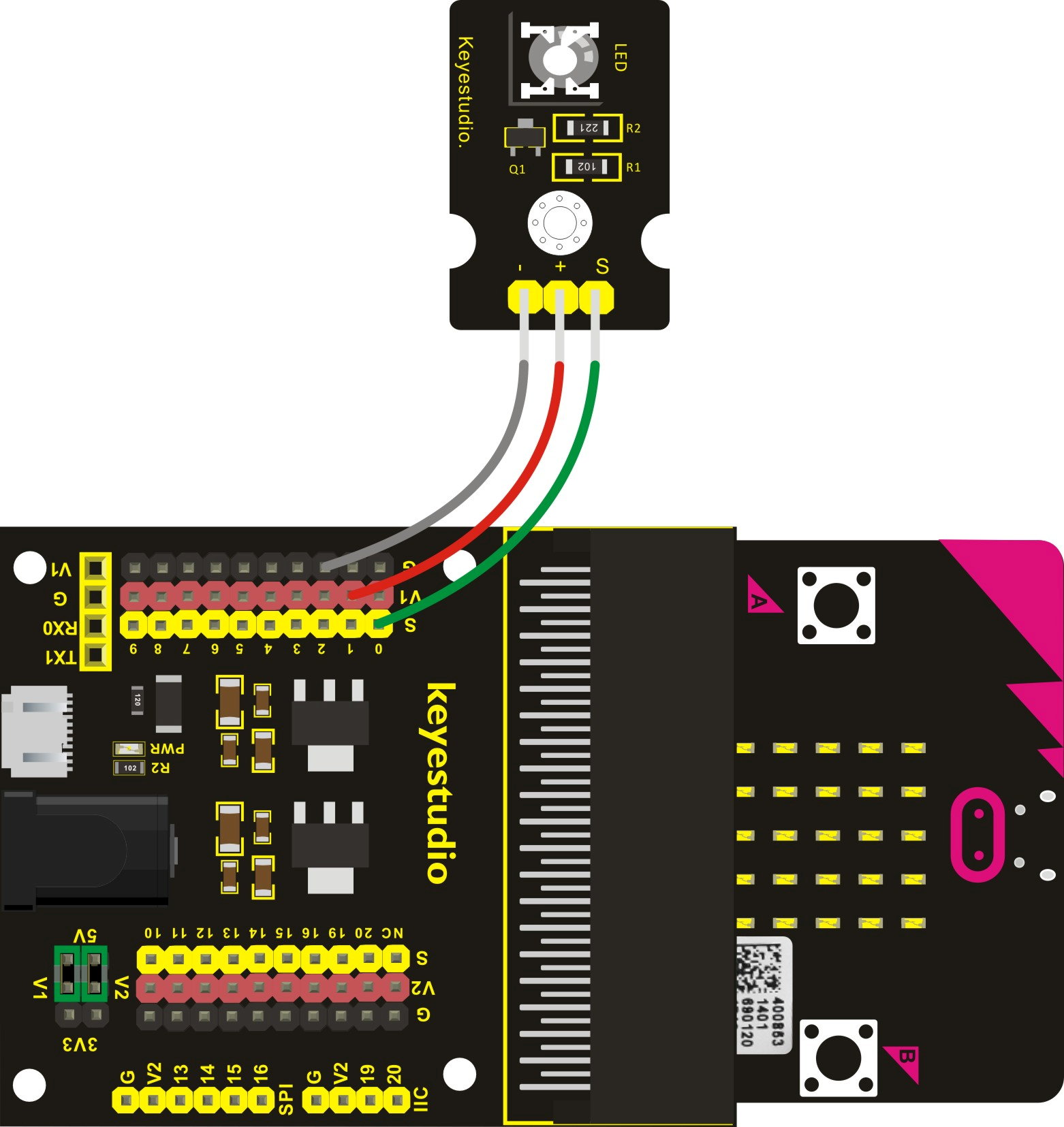
Software
Start a New Project
and let’s call it “Conditionals”.
We are going to make a lantern that changes brightness when we press a button. We will turn on, turn off, and control the brightness with only 1 button!
Let’s use what we know from Analog Out and create a program
that writes 1023
to P0
:
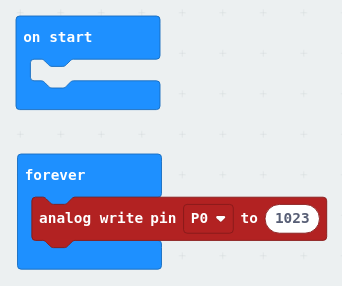
analog write pin
Code Block
Next, we will change the brightness to 0
when we press button A
:
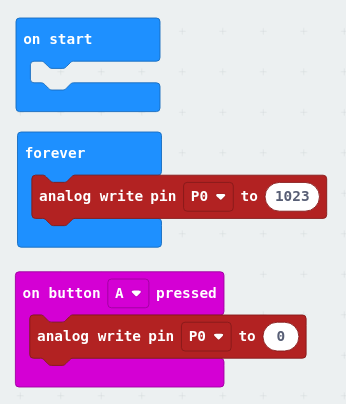
button A
Code Block
Let’s try the simulation… hmmm… it is not working???
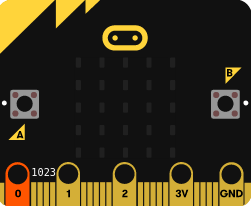
Why is it not working? When we press button A
, we change the value of P0
to
0
for a split second. However, the code in the forever
block gets activated
as soon as we pressed the button, which returns the value of P0
to 1023
.
This is where variables come to the rescue!
We are going to save the value of brightness into a variable, so it doesn’t change suddenly.
Let’s create a variable
called brightness
. We are going to click on Make a Variable...
from the Variables
blocks.
variable
Code Block
new variable
will be called brightness
.
You will see three new blocks. With the top most block, you can read the value of the variable. The middle one is to set the value of the variable to a specific number. The third one is to change the value by a certain amount.
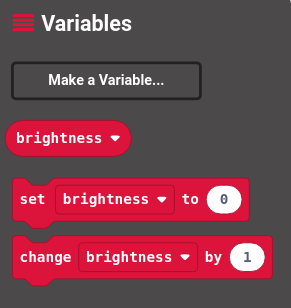
Let’s grab our new blocks and place them in our code. First, when we press
button A
, we are going to set brightness
to 0
.
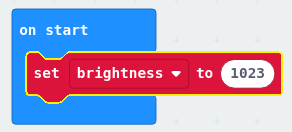
brightness
to 0
.
Then, we need to read the value of brightness
in our forever
block, so
we know which brightness to use.
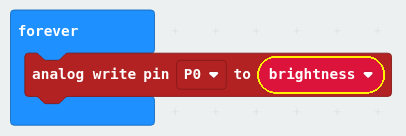
brightness
.
P0
will be
always 0
! We need to set the initial value of the variable!
Finally, we will set the initial value of brightness
to 1023
. We do
this by using the block on start
.
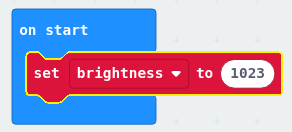
brightness
to 1023
.
We now have something like this:
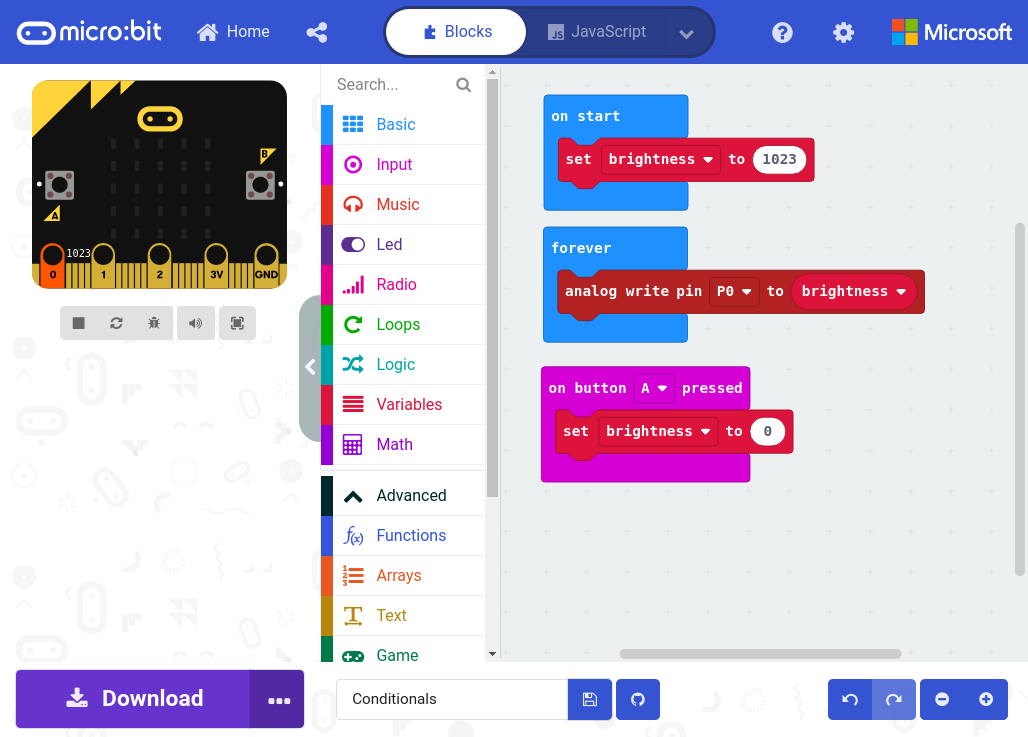
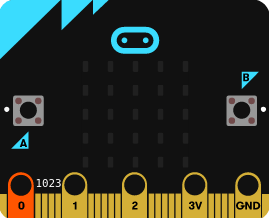
Changing the brightness
Our program only works once. This is no good. How can we make it toggle the LED?
Here, we need to use conditionals. We are going to use the if <true▼> then (+)
code block from Logic
blocks.
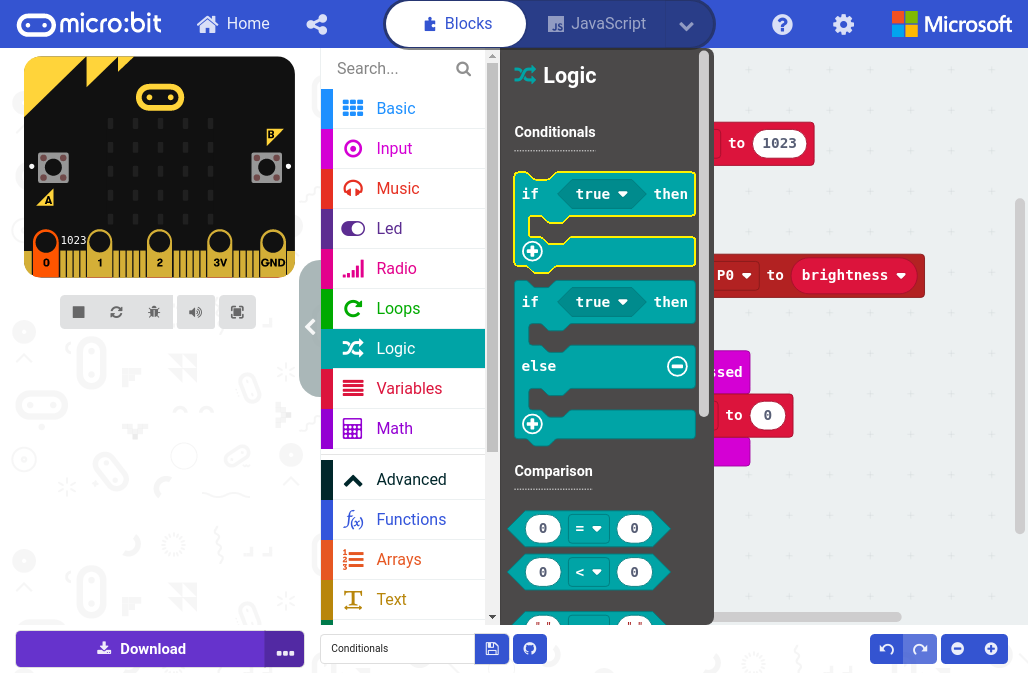
if
Code Block
We are going to set the brightness
to 0
if its value is 1023
,
otherwise, we set it to 1023
.
To do that, drag and drop the code block inside on button [A▼] pressed
,
and move the set
block inside this conditional:
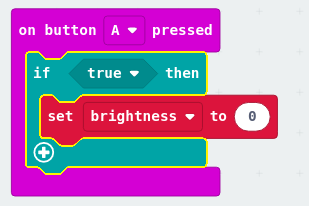
Then, click on the (+)
button and let’s add a second set
:
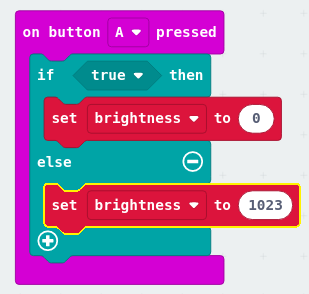
Finally, we are going to add the condition using the comparison
code block from
Logic
blocks.
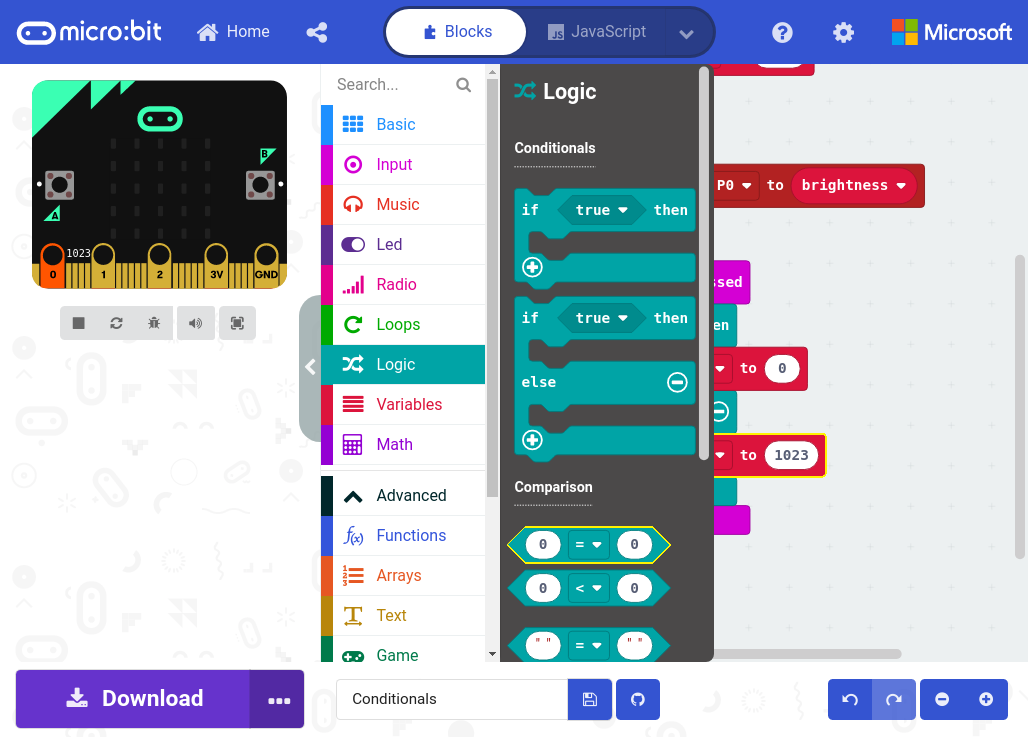
Drag and drop the first one <(0) [=▼] (0)
> inside the <true▼>
slot of the
conditional:
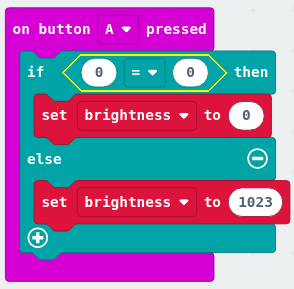
Now, we just compare if brightness
is equal to 1023
:
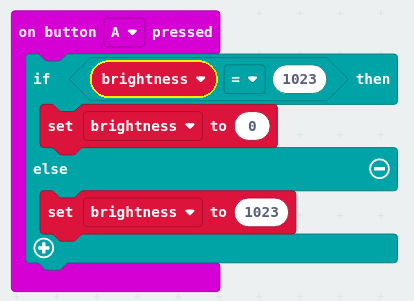
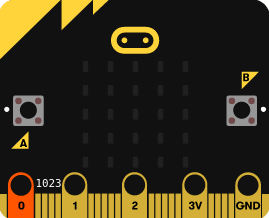
Are you up for a challenge?
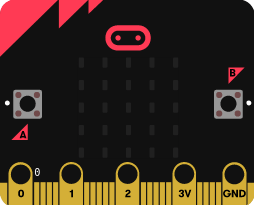
Try to make a program that turns on the LED when you press button A
,
turns off the LED when pressing the same button and can cycle through different
brightness with the same button.
Once you finish, you can check your solution with the answer here.