Analog Out
Code blocks used in this tutorial:
Analog Signals
In electronics, there are two types of signals: digital and analog.
A digital signal only has two states: on
or off
.
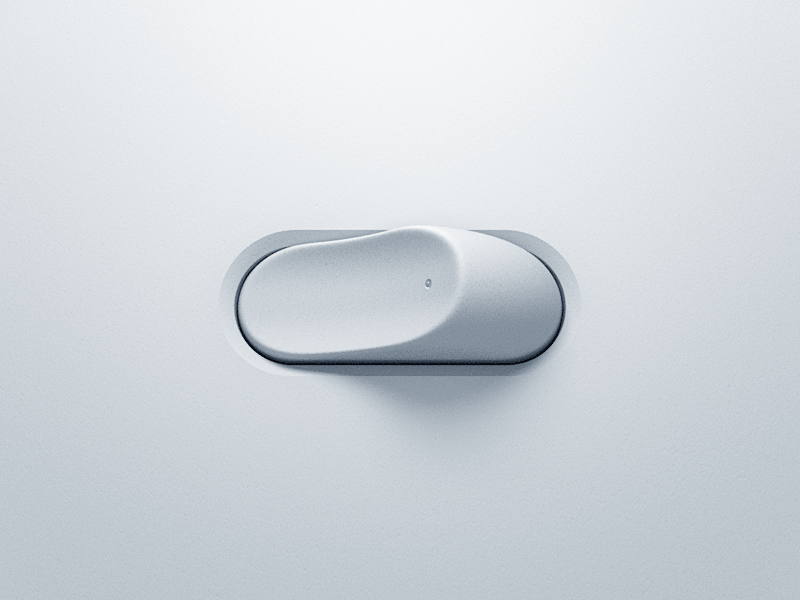
An analog signal can have multiple states within a range (from 0
to 1023
).

0~1023
is the digital representation of an analog signal? It represents a 10-bit
(2^10
) resolution signal. 2^10
= 1024
values!
In this tutorial, we are going to learn how to control analog signals.
Electronics
For this tutorial, we are going to use the expansion pack
, the LED module
and 3 cables.
Let’s do the following steps:
- Grab 1 cable and connect the
S
pin of theLED module
to theS0
pin of theexpansion pack
. Make sure that the cable is connected to theS0
position. - Next, grab another cable and connect the
+
pin of theLED module
to theV1
pin of theexpansion pack
. This can be connected on any pin as long as it is a red pin ofV1
. - Finally, grab the last cable and connect the
-
pin of theLED module
to theG
pin of theexpansion pack
. This can be connected on any pin as long as it is a black pin ofG
.
You should have something like the picture below:
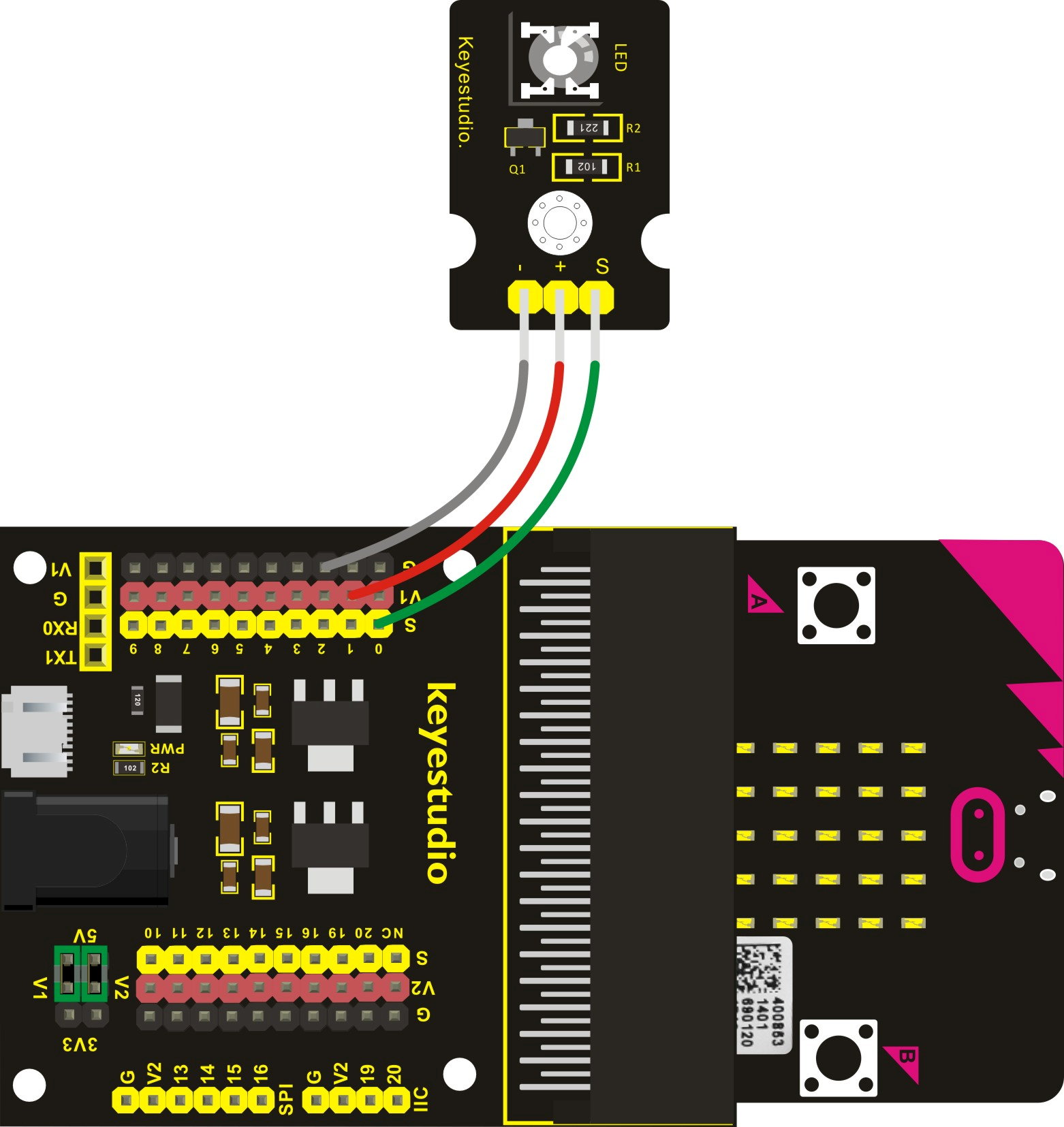
Software
Start a New Project
and let’s call it “AnalogOut”.
To control analog signals, we are going to use the code block analog write pin [P0▼] to (1023)
from the Advanced
> Pins
blocks.

analog write pin
Code Block
By default, this block is set to 1023
, which means “fully on”. If you
change this number to 0
, it means “fully off”. Any value in between will
change the brightness of the LED.
Let’s make a simple dimming LED animation that goes from “fully off” to
25%, 50%, 75% brightness, and “fully on”, with a 500ms
pause between states.
Hint!
Before we can code the analog value, first we need to calculate their values. We
know that “fully off” (or 0%
) is 0
and “fully on” (or 100%
) is 1023
. What about the other
numbers?
We can use the Rule of Three
(a.k.a. Cross-multiplication
) to
calculate their values.
If 100%
is to 1023
, then 25%
is to x
. Then, we can solve for x
:
$$x_{25} = \frac{1023}{100}*25 = 255.75 \approx 255$$
We do the same for 50%
and 75%
.
$$x_{50} = \frac{1023}{100}*50 = 511.5 \approx 511$$ $$x_{75} = \frac{1023}{100}*75 = 767.25 \approx 767$$
Finally, you should have something like this inside the forever
block:

The simulation should show something like this. Notice how pin 0
changes
color gradually. That means different voltages are flowing through the pin.

Now, upload the code and see it in action!
Are you up for a challenge?
Try to make a program that turns on the LED when you press button A
and
turns off the LED when you press button B
. However, if you press A + B
, the
LED changes to 50%
brightness.
Once you finish, you can check your solution with the answer here.